Cybersecurity Essentials for Fullstack Developers
18 Feb, 2025
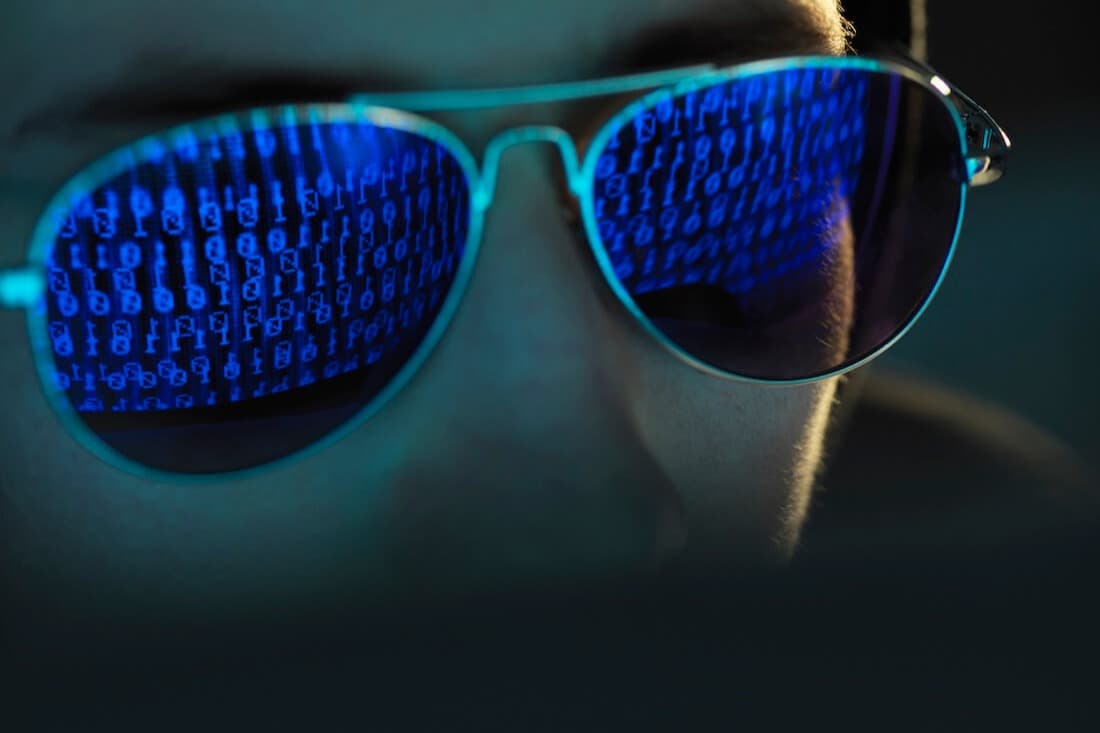
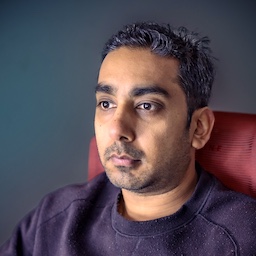
Sumit Govil
Founder, Allevio Soft
Cybersecurity in fullstack development is all about protecting applications from hackers, data breaches, and security threats across the entire technology stack. This includes the frontend (what users interact with), the backend (server-side logic), the database (where data is stored), and even the network that connects everything.
A secure application ensures three key things:
Confidentiality: Sensitive information is only accessible to the right people.
Integrity: Data remains unchanged and trustworthy.
Availability: The application and its data are accessible when needed.
Many developers focus only on writing functional code and overlook security. However, an insecure application can be worse than a broken one, as it can lead to serious consequences such as data theft, unauthorized access, or financial loss.
Why Security is a Shared Responsibility
Security is not just the job of cybersecurity experts or the security team. Every fullstack developer must think about security from the very beginning of development. If one part of the stack is weak, the entire application is at risk.
Frontend Security (Client-Side Protection) | Preventing Cross-Site Scripting (XSS): Attackers inject malicious scripts into web pages viewed by users. Stopping Cross-Site Request Forgery (CSRF): Protecting users from unwanted actions triggered by malicious websites. Clickjacking Defense: Preventing malicious sites from embedding your site in an invisible iframe and tricking users into clicking harmful buttons. |
Backend Security (Server-Side Protection) | Securing APIs and authentication mechanisms to prevent unauthorized access. Implementing proper access control and authorization to ensure users only access what they are allowed to. Protecting against SQL Injection, where attackers manipulate database queries to steal or modify data. |
Database Security | Using parameterized queries to prevent attackers from injecting harmful SQL commands. Enforcing least privilege access, ensuring users have only the necessary permissions to interact with the database. Encrypting sensitive data at rest (stored) and in transit (during transmission) to prevent leaks. |
DevSecOps Integration (Security in Deployment) | Adding automated security tests in Continuous Integration/Continuous Deployment (CI/CD) pipelines. Regularly scanning dependencies and third-party libraries for vulnerabilities. Enabling logging and monitoring to detect and respond to threats in real time. |
A secure application is built from day one, and not as an afterthought. Security must be integrated into every step of development.
Common Cybersecurity Myths (and Why They’re Wrong!)
Many developers fall for common security misconceptions, which can lead to poor security decisions.
🚫 “Security is only the security team’s job.”
✅ Wrong! Developers write the code that attackers exploit. If security isn’t considered from the start, even the best security team can’t fully protect the application.
🚫 “HTTPS alone makes my site secure.”
✅ Partially true, but not enough! HTTPS encrypts data in transit, but it does not protect against attacks like XSS, SQL Injection, or misconfigured API security.
🚫 “Using a framework means my code is secure.”
✅ Not always! While frameworks (like React, Express, Django) come with security features, developers must still write secure code and follow best practices.
Understanding Threats and Vulnerabilities
Before we can protect our applications, we need to understand what we’re up against. Cybersecurity threats evolve constantly, but many attacks follow predictable patterns. Fullstack developers must be aware of these risks to build applications that are secure by design.
OWASP Top 10 Vulnerabilities
The OWASP (Open Web Application Security Project) Top 10 is a well-known list of the most critical web application security risks. It serves as a must-know for every fullstack developer.
Vulnerability | How to prevent? ✅ |
---|---|
Broken Access Control Attackers gain unauthorized access to data or functionality due to poor permissions | Enforce role-based access control (RBAC) and least privilege access. Deny access by default and grant permissions explicitly. Regularly test authorization logic to ensure users can only access what they should. |
Cryptographic Failures Sensitive data (like passwords or credit card info) is stored or transmitted without proper encryption, making it easy to steal | Use TLS (HTTPS) for all communications. Store passwords only as hashed and salted values (bcrypt, Argon2). Never hardcode secrets in code, use environment variables or secret management tools. |
Injection Attacks (SQL, NoSQL, LDAP, XSS, RCE) Attackers inject malicious input into a query or command to execute unintended actions | Use parameterized queries and ORMs to prevent SQL Injection. Escape and sanitize user input before rendering it in the frontend (against XSS). Use input validation and strict typing to prevent malicious data execution |
Insecure Design Applications are built without security in mind, making them inherently vulnerable | Security should be part of the development process (Shift Left Security) Conduct threat modeling before development to identify weak points. Implement secure coding practices from the beginning. |
Security Misconfiguration Developers leave default settings enabled, expose stack traces, or forget to remove debugging tools, making it easy for hackers to find vulnerabilities | Disable default accounts and change system defaults. Remove debugging logs, error messages, and unnecessary services in production. Implement secure headers (e.g., X-Frame-Options, Content-Security-Policy). |
Vulnerable Components Using outdated libraries, plugins, or dependencies exposes your app to known exploits | Regularly update dependencies and check for vulnerabilities using tools like Snyk or Dependabot. Avoid using unmaintained open-source libraries. Remove unused dependencies from the codebase. |
Identification & Authentication Failures Weak passwords, exposed session tokens, or improper authentication allow attackers to impersonate users | Use Multi-Factor Authentication (MFA) whenever possible. Hash passwords using bcrypt or Argon2. Never store plain text passwords! Implement session timeouts and secure logout flows. |
Software & Data Integrity Failures Attackers modify software updates or inject malicious code into your application’s supply chain | Use signed and verified updates for dependencies. Protect Continuous Integration/Continuous Deployment (CI/CD) pipelines from unauthorized modifications. Implement checksum verification when downloading third-party software |
Security Logging & Monitoring Failures Many companies don’t detect breaches until it’s too late because they don’t log or monitor suspicious activities | Implement centralized logging and alert systems to track security events. Regularly audit access logs to detect anomalies. Enable real-time security monitoring with tools like ELK Stack, Splunk, or AWS CloudTrail. |
Server-Side Request Forgery (SSRF) Attackers trick the server into making requests to internal systems, gaining access to sensitive internal resources | Validate and sanitize URLs that are accepted from user input. Block internal requests unless explicitly required. Implement network segmentation and firewall rules to limit internal system exposure. |
Common Attacks & Real-World Examples
Now that we understand the top vulnerabilities, let’s look at how some of these attacks work in real life.
Common Attacks | ✅ Prevention |
---|---|
SQL Injection (SQLi) Attackers inject malicious SQL queries to gain unauthorized access to data. SELECT * FROM users WHERE username = 'admin' OR '1'='1'; | Always use prepared statements and parameterized queries: db.query("SELECT * FROM users WHERE username = ?", [userInput]); |
Cross-Site Scripting (XSS) An attacker injects malicious JavaScript into a web page, which then executes in a user’s browser. <input type="text" value="<script>alert('Hacked!')</script>"> If this input is not sanitized, it can execute and steal cookies or redirect users to malicious websites. | Use Content Security Policy (CSP) to limit script execution. Escape and sanitize user input before rendering in the frontend. |
Cross-Site Request Forgery (CSRF) Attackers trick users into unintentionally performing actions on a site where they’re logged in. A hidden form on a malicious site can automatically transfer money from a logged-in user’s account: <form action="https://bank.com/transfer" method="POST"> <input type="hidden" name="amount" value="5000"> <input type="hidden" name="to" value="attacker_account"> <input type="submit"> </form> If the user is logged in, their session automatically submits the request. | Use CSRF tokens to validate legitimate requests. Require re-authentication before critical actions |
Frontend Security Best Practices
Frontend security is often overlooked because many developers assume “hackers attack the backend.” But in reality, the frontend is the first point of contact for users, and attackers exploit it to inject malicious scripts, steal data, or manipulate user sessions.
A secure frontend ensures:
✅ Users are protected from phishing, cross-site scripting (XSS), and session hijacking.
✅ Data integrity is maintained by preventing unauthorized access to browser storage.
✅ Backends remain secure by blocking harmful requests before they even reach the server.
Let’s break down essential security practices every fullstack developer should follow.
Secure Handling of User Inputs
Any input from a user can be a potential attack vector if not properly handled. Whether it’s a search bar, login form, or comment box, never trust user input!
🚨 Why is this important?
Hackers inject malicious JavaScript, SQL queries, or remote code into forms or URLs to steal data or exploit vulnerabilities.
✅ Best Practices
🔹 Sanitize & Escape Input: Remove or encode potentially dangerous characters before rendering them.
For HTML sanitization, use libraries like DOMPurify (for React/Vanilla JS).
import DOMPurify from 'dompurify';
const safeHTML = DOMPurify.sanitize(userInput);
For escaping JavaScript/HTML characters, use:
function escapeHTML(str) { return str.replace(/[&<>"']/g, (match) => ({ '&': '&', '<': '<', '>': '>', '"': '"', "'": ''', }[match])); }
🔹 Validate Input on Both Frontend & Backend:
Frontend validation improves UX (e.g., restricting password length).
Backend validation is critical as attackers can bypass frontend validation using tools like Postman or Burp Suite.
Content Security Policy (CSP) - Defense Against XSS Attacks
Cross-Site Scripting (XSS) is one of the most common frontend attacks. Attackers inject scripts that run on users’ browsers, stealing data or hijacking sessions.
🚨 Why is CSP important?
Without Content Security Policy (CSP), a single XSS vulnerability can allow attackers to execute malicious scripts on your site.
✅ How to Prevent XSS with CSP?
A CSP header tells browsers which scripts are allowed to run on your site.
🔹 Basic CSP Header (Block External Scripts)
Content-Security-Policy: default-src 'self'; script-src 'self'
• default-src 'self' → Allows content only from your own domain.
• script-src 'self' → Blocks all third-party JavaScript (except explicitly allowed sources).
🔹 CSP Header with Hash for Allowed Scripts
Content-Security-Policy: default-src 'self'; script-src 'self' 'sha256-abc123'
• This allows only scripts with the exact hash (sha256-abc123), blocking all inline scripts unless explicitly authorized.
🔹 How to Implement CSP in Next.js / Express?
For Next.js: Add security headers in next.config.js:
module.exports = { async headers() { return [ { source: "/(.*)", headers: [ { key: "Content-Security-Policy", value: "default-src 'self'; script-src 'self' 'sha256-abc123'", }, ], }, ]; }, };
For Express.js: Add CSP headers using helmet:
const helmet = require('helmet'); app.use(helmet.contentSecurityPolicy({ directives: { defaultSrc: ["'self'"], scriptSrc: ["'self'", "'sha256-abc123'"], } }));
Secure Cookies & CORS Best Practices
🚨 Why is this important?
Cookies store session tokens. If stolen, an attacker can impersonate a user.
CORS (Cross-Origin Resource Sharing) defines which websites can access your API. Misconfigurations can allow unauthorized data leaks.
✅ Best Practices
🔹 Secure Cookie Settings (Prevent Session Hijacking)
Set cookies with HttpOnly, Secure, and SameSite=Strict flags to prevent XSS and CSRF attacks.
res.cookie('sessionId', token, { httpOnly: true, // Prevents JavaScript from accessing cookies secure: true, // Ensures cookies are only sent over HTTPS sameSite: 'Strict', // Blocks CSRF attacks });
🔹 Restrict CORS (Cross-Origin Requests)
CORS controls which websites can fetch data from your API. Avoid Access-Control-Allow-Origin: *. It exposes your API to any attacker!
✅ Correct way:
const cors = require('cors'); app.use(cors({ origin: "https://your-trusted-site.com", // Only allow specific origins credentials: true, }));
🔹 Avoid Storing Sensitive Data in localStorage/sessionStorage
🚫 Why? Data in localStorage is accessible via JavaScript, making it vulnerable to XSS attacks.
✅ Alternative: Store sensitive data in HTTP-only cookies instead of localStorage.
Preventing Clickjacking
🚨 What is Clickjacking?
Clickjacking tricks users into clicking invisible elements, unknowingly performing actions (e.g., transferring money, liking a post, or granting permissions).
✅ How to Prevent Clickjacking?
🔹 Use X-Frame-Options to Block Unauthorized Embedding
X-Frame-Options: DENY
• DENY: Blocks the site from being embedded in an iframe (recommended).
• SAMEORIGIN: Allows embedding only from the same origin.
🔹 Use CSP’s frame-ancestors Directive
Content-Security-Policy: frame-ancestors 'none';
This is a modern alternative to X-Frame-Options, preventing any site from embedding yours.
🔹 How to Implement in Next.js / Express?
For Next.js:
module.exports = { async headers() { return [ { source: "/(.*)", headers: [ { key: "X-Frame-Options", value: "DENY" }, { key: "Content-Security-Policy", value: "frame-ancestors 'none'" }, ], }, ]; }, };
For Express.js:
const helmet = require('helmet'); app.use(helmet.frameguard({ action: 'deny' }));
Backend Security Best Practices
While frontend security protects users, backend security is the last line of defense. If an attacker gains access to your backend, they can steal user data, modify records, or even take full control of your application.
A secure backend ensures:
✅ Only authenticated users access protected resources.
✅ Data remains safe from injection attacks.
✅ Bots and automated scripts are blocked from overloading your server.
✅ Sensitive credentials are kept private.
Secure API Endpoints
APIs are the backbone of modern applications, but they’re also prime targets for attackers. An unprotected API can expose sensitive user data to unauthorized parties.
🚨 Common API Security Threats
• Unauthorized access – Attackers call APIs they shouldn’t have access to.
• Leaked API keys – Exposing keys in frontend JavaScript allows anyone to use them.
• Excessive privileges – APIs return more data than needed, increasing exposure.
✅ Best Practices for API Security
🔹 Use Secure Authentication (JWT, OAuth, API Keys)
• JWT (JSON Web Tokens) are commonly used for authentication:
const token = jwt.sign({ userId: user.id }, process.env.JWT_SECRET, { expiresIn: '1h' });
• OAuth 2.0 is recommended for third-party authentication (e.g., Google Sign-In).
• API Keys should be stored securely and never exposed on the frontend.
🔹 Implement Role-Based Access Control (RBAC)
Not every user should have access to all API endpoints. Use RBAC to restrict permissions.
const checkRole = (role) => (req, res, next) => { if (req.user.role !== role) return res.status(403).send("Forbidden"); next(); };
// Protect an admin route app.get('/admin', checkRole('admin'), (req, res) => { res.send("Welcome, Admin!"); });
🔹 Use HTTPS for API Requests
Always enforce HTTPS to encrypt data in transit. Reject HTTP requests to prevent man-in-the-middle (MITM) attacks.
app.use((req, res, next) => { if (!req.secure) { return res.redirect(`https://${req.headers.host}${req.url}`); } next(); });
Preventing SQL Injection
SQL Injection (SQLi) is one of the most dangerous web attacks. It allows attackers to manipulate database queries and steal data.
🚨 How SQL Injection Works
An attacker enters a malicious query:
SELECT * FROM users WHERE email = 'admin' OR '1'='1'
Since '1'='1' is always true, this query bypasses authentication and logs in the attacker as admin!
✅ Best Practices to Prevent SQL Injection
🔹 Use Parameterized Queries (Prepared Statements)
Never concatenate user input in queries. Always use placeholders:
db.query("SELECT * FROM users WHERE email = ?", [email]);
🔹 Use ORM Libraries (Object-Relational Mappers)
ORMs like Sequelize, Prisma, or Mongoose abstract database queries, reducing the risk of SQL Injection.
Example using Prisma:
const user = await prisma.user.findUnique({ where: { email: req.body.email }, });
🔹 Sanitize and Validate User Input
Ensure only valid data is accepted:
const email = req.body.email.trim().toLowerCase(); if (!validator.isEmail(email)) { return res.status(400).send("Invalid email format"); }
Rate Limiting & Bot Protection
Attackers use bots to flood your API with requests, causing denial of service (DoS) attacks or attempting brute-force logins.
🚨 Why Rate Limiting Matters
Without rate limiting, an attacker could:
🔴 Try millions of passwords in seconds (brute force).
🔴 Spam APIs with fake requests (DoS attack).
✅ Best Practices for Rate Limiting & Bot Protection
🔹 Limit Requests per IP using express-rate-limit
const rateLimit = require("express-rate-limit");
const apiLimiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100, // limit each IP to 100 requests per window message: "Too many requests, please try again later.", });
app.use("/api", apiLimiter);
🔹 Use CAPTCHA for Forms
Protect login, signup, and contact forms from bots by integrating Google reCAPTCHA.
<form action="/login"> <div class="g-recaptcha" data-sitekey="your-site-key"></div> </form> <script src="https://www.google.com/recaptcha/api.js"></script>
🔹 Detect and Block Bots
Use user-agent analysis and request behavior tracking to block bots.
Secure Environment Variables
Many developers accidentally expose sensitive credentials in public repositories. Hackers actively scan GitHub for .env files containing secrets like:
❌ API keys
❌ Database passwords
❌ JWT secrets
🚨 Real-World Example: A Leaked API Key
A developer accidentally commits this to GitHub:
DATABASE_URL=mongodb+srv://username:password@cluster.mongodb.net
Hackers immediately scan GitHub for leaked keys and steal sensitive data before the mistake is noticed.
✅ Best Practices for Secure Environment Variables
🔹 Use .env Files, But NEVER Commit Them!
1. Store credentials in .env:
DB_PASSWORD=mysecurepassword
2. Load environment variables using dotenv:
require('dotenv').config(); const dbPassword = process.env.DB_PASSWORD;
🔹 Add .env to .gitignore
Ensure Git ignores sensitive files to prevent accidental commits.
# .gitignore .env
🔹 Use Secrets Management Tools (For Production)
For large-scale applications, store secrets in:
✅ AWS Secrets Manager
✅ Google Cloud Secret Manager
✅ HashiCorp Vault
Example: Fetching a secret from AWS Secrets Manager
const AWS = require('aws-sdk'); const secretsManager = new AWS.SecretsManager();
async function getSecret(secretName) { const secret = await secretsManager.getSecretValue({ SecretId: secretName }).promise(); return JSON.parse(secret.SecretString); }
Database Security Best Practices
Databases store sensitive user data, making them one of the biggest targets for hackers. If an attacker gains access to your database, they can steal personal information, modify records, or delete everything.
A secure database ensures:
✅ Only authorized users can access it.
✅ Data remains confidential through encryption.
✅ Injection attacks are blocked before they reach the database.
Let’s explore essential database security best practices every fullstack developer should follow.
Securing SQL & NoSQL Databases
SQL and NoSQL databases have different architectures, but both require strong security measures to prevent data breaches.
🚨 Common Database Security Risks
🔴 Unprotected remote access – Attackers connect to the database from the internet.
🔴 Overprivileged accounts – Users have more permissions than needed.
🔴 Unencrypted data – If stolen, data can be read in plain text.
✅ Best Practices for SQL & NoSQL Security
🔹 Disable Remote Database Access Unless Necessary
• Allow only trusted IPs to connect to the database.
• Use firewall rules to block public access.
🔹 Follow the Least Privilege Principle (Role-Based Access Control - RBAC)
• Grant only the permissions required for each user.
• Avoid using the root/admin account for regular queries.
• In PostgreSQL/MySQL, create restricted user roles:
CREATE USER app_user WITH PASSWORD 'securepassword'; GRANT SELECT, INSERT ON users TO app_user;
🔹 Encrypt Sensitive Data
• Use AES-256 encryption to store sensitive fields securely.
• Never store passwords in plain text. Use bcrypt hashing instead.
NoSQL Injection Prevention
NoSQL Injection is similar to SQL Injection, but it targets NoSQL databases like MongoDB, Firebase, and CouchDB.
🚨 How NoSQL Injection Works
An attacker modifies an input to manipulate the query logic.
💥 Example (MongoDB NoSQL Injection)
// Vulnerable query User.findOne({ username: req.body.username }).exec();
If an attacker sends:
{ "username": { "$gt": "" } }
It bypasses authentication and logs in without credentials!
✅ How to Prevent NoSQL Injection
🔹 Validate & Sanitize Input
• Reject unexpected data types before sending queries.
• Use libraries like Joi or Yup for input validation.
✅ Example using Joi validation
const Joi = require('joi');
const schema = Joi.object({ username: Joi.string().alphanum().min(3).max(30).required() });
const { error } = schema.validate(req.body); if (error) return res.status(400).send(error.details[0].message);
🔹 Use Parameterized Queries Instead of Direct User Input
✅ Safe way to query MongoDB using Mongoose
User.findOne({ username: sanitize(req.body.username) }).exec();
🔹 Limit Query Depth in GraphQL APIs
• Prevent overly deep queries that could be used for data scraping attacks.
• Use GraphQL depth-limiting middleware.
Data Encryption Best Practices
Even if an attacker breaches the database, proper encryption ensures stolen data remains unreadable.
✅ Best Practices for Encryption
🔹 Encrypt Data at Rest (Stored Data)
• Use Transparent Data Encryption (TDE) to encrypt databases without modifying queries.
• In PostgreSQL, enable encryption with:
ALTER SYSTEM SET data_encryption = 'on';
🔹 Encrypt Data in Transit (During Transmission)
• Use SSL/TLS to encrypt database connections.
• In MongoDB, enable TLS with:
net: ssl: mode: requireSSL PEMKeyFile: /etc/ssl/mongodb.pem
🔹 Never Store Passwords. Store Hashed Versions
• Use bcrypt or Argon2 to securely hash passwords.
• Example using bcrypt in Node.js:
const bcrypt = require('bcrypt'); const hashedPassword = await bcrypt.hash(userPassword, 10);
🔹 Use Key Management Systems (KMS)
• Store encryption keys separately using AWS KMS or HashiCorp Vault.
• Never hardcode encryption keys in the code!
Conclusion
Cybersecurity is not a one-time task. It's a continuous process that evolves with new threats. As a fullstack developer, security should be a mindset, not an afterthought
Security is a shared responsibility across frontend, backend, database, and deployment layers. Even a small vulnerability can lead to massive breaches, so it’s always better to proactively secure your applications rather than fixing issues later.
By following these best practices, you’re not just writing code, you’re building resilient, trustworthy applications that keep user data safe.