Level Up Your Search Game with AI-Powered Search for Full-Stack Apps
28 Feb, 2025
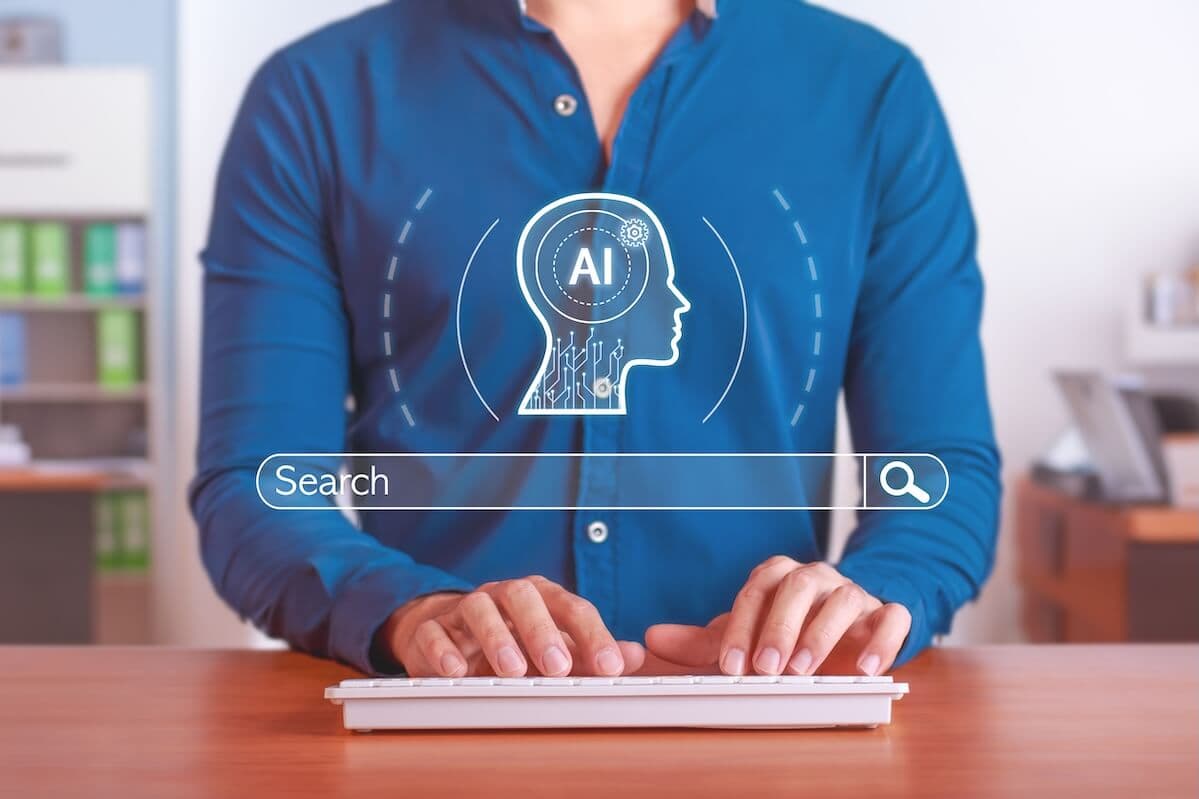
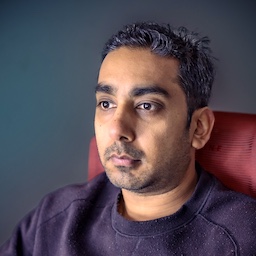
Sumit Govil
Founder, Allevio Soft
Imagine trying to find something specific, like:
💬 “I need a laptop with a long battery life for video editing under $1500.”
💬 “Find me a pediatrician in New York who accepts Medicare and has good reviews.”
A basic keyword-based search might return random laptops or any doctor in New York, instead of truly understanding what you’re looking for.
When people search for something online, they expect quick and accurate results. Whether they’re looking for a job, a doctor, a lawyer, or a specific product, traditional search systems often fail to deliver relevant results because they rely too much on exact keyword matching.
Most traditional search engines ignore context, don't rank results intelligently, or force users to filter manually.
To understand this better, let’s take an example of a home services platform where users look for electricians, plumbers, or landscapers.
💬 “I need a licensed electrician to fix my wiring this Saturday.”
💬 “Find me a reliable plumber for an emergency leak in Sydney.”
How does a traditional search system handle these queries?
❌ It only matches keywords like “electrician” or “plumber” without understanding availability, licensing, or urgency.
❌ It forces users to filter manually by selecting days of work, location, and insurance status.
❌ It doesn’t prioritize urgency. An emergency job request is treated the same as a general inquiry.
We need a smarter, AI-powered search system that can:
âś… Understand natural language queries (instead of relying on keywords).
âś… Match based on meaning (not just exact words).
âś… Rank providers based on availability, urgency, and other real-world factors.
That’s exactly what we are going to build today. But first, let's understand how AI steps up this game.
How AI Can Make Search Smarter
Traditional search systems struggle because they rely on simple keyword matching. But what if search could be as smart as a human assistant? That’s where AI-powered search comes in.
Instead of just looking for matching words, AI can understand the meaning behind a query. This means a user can type:
💬 “I need a gardener in Melbourne who can mow my lawn and do soil top-up this Saturday.”
And AI will break it down like this:
âś… Service Type: Gardening
âś… Tasks Needed: Lawn mowing, soil top-up
âś… Location: Melbourne
âś… Availability: This Saturday
AI-powered search works using a combination of :
Natural Language Processing (NLP): Helps AI understand full sentences and extract important details like location, date, and service type.
Vector Search (Embeddings): Converts words and sentences into numbers (vectors) so the system can compare and rank results based on meaning, not just keywords.
Smart Ranking: AI can prioritize results based on availability, licensing, customer reviews, and urgency, making search more relevant.
AI powered search is better as:
Users don’t need to type “Electrician + Sydney + Saturday.” They can just type what they want naturally.
AI finds relevant results, even if the user’s words don’t match exactly with what’s in the database.
No need to go through multiple filters and dropdowns. The right service providers show up instantly.
With AI, search becomes smarter, faster, and more user-friendly leading to better experiences for users and more business for service providers.
Setting Up the Foundation
The first step is choosing the right database. Since we need to store service providers’ details and perform AI-powered search, we need a database that supports:
Fast search queries
Handling structured data (services, location, availability, etc.)
AI-driven ranking based on meaning, not just keywords
PostgreSQL is a powerful, open-source database that supports AI-based search using PGVector, an extension that allows us to store and search AI-generated embeddings (vector representations of text).
🔹 Handles complex search queries - It lets us search for service providers using semantic meaning, rather than just exact words.
🔹 Supports AI indexing (PGVector) - This allows us to store embeddings (AI-generated representations of text) and search them efficiently.
🔹 Scalable & Reliable - Works well for large datasets and can handle thousands of queries per second.
Creating the Service Providers Table
We need to store details like name, services, location, availability, and insurance. Plus, we’ll add a VECTOR column to store AI-generated embeddings.
CREATE TABLE service_providers ( id SERIAL PRIMARY KEY, name TEXT, services TEXT[], -- Example: ["Lawn Mowing", "Soil Top-Up"] location TEXT, -- Example: "Melbourne" work_days TEXT[], -- Example: ["Saturday", "Sunday"] has_insurance BOOLEAN, description TEXT, embedding VECTOR(1536) -- Stores AI-generated vector representation );
So this is what we are doing here:
✔ Storing provider details – Name, services, location, availability, and insurance.
✔ Adding an embedding column – This is where we will store AI-generated vector data for each provider.
✔ Using PostgreSQL’s array types – This helps us store multiple values (e.g., available work days) in a single field.
Enable PGVector for AI Search:
Before we move to the next step, let’s activate the PGVector extension:
CREATE EXTENSION IF NOT EXISTS vector; CREATE INDEX idx_embedding ON service_providers USING ivfflat (embedding);
This sets up our database to handle AI-powered search efficiently.
Generating AI Embeddings for Service Providers
Now that we’ve set up our PostgreSQL database, the next step is to convert service provider data into AI-readable format. This process is called embedding generation.
Embeddings are numerical representations of text. Instead of storing text as plain words, AI converts it into a vector (a list of numbers) that captures the meaning of the text.
For example, these two sentences:
💬 “Find me a gardener for lawn mowing.”
💬 “Looking for someone to cut my grass.”
Both mean the same thing, but a keyword-based search won’t match them. However, with embeddings, AI understands that both sentences are similar, even if the words are different.
Generate Embeddings
We will use OpenAI’s Embeddings API to convert each service provider’s details (name, services, location, description) into embeddings. These embeddings will then be stored in PostgreSQL for fast retrieval during search.
Let’s write a Node.js script to fetch provider details, generate embeddings, and store them in the database.
const { OpenAI } = require("openai"); const { Client } = require("pg"); require("dotenv").config();
const openai = new OpenAI({ apiKey: process.env.OPENAI_API_KEY }); const db = new Client({ connectionString: process.env.DATABASE_URL });
async function getEmbedding(text) { const response = await openai.embeddings.create({ model: "text-embedding-ada-002", input: [text] }); return response.data[0].embedding; // Return the embedding (vector representation) }
async function storeEmbeddings() { await db.connect(); const result = await db.query("SELECT id, name, services, location, description FROM service_providers");
for (const provider of result.rows) { const textData = `${provider.name} ${provider.services.join(", ")} ${provider.location} ${provider.description}`; const embedding = await getEmbedding(textData);
await db.query("UPDATE service_providers SET embedding = $1 WHERE id = $2", [embedding, provider.id]); } await db.end(); console.log("Embeddings stored successfully!"); }
storeEmbeddings();
Here, we are:
âś” Fetching all service provider records from PostgreSQL.
âś” Combining name, services, location, and description into a single text input.
✔ Generating an embedding (vector) for each provider using OpenAI’s Embeddings API.
âś” Updating the PostgreSQL database with the generated embeddings.
And this is important because of following reasons:
A query like “Find someone to trim my hedges” will match a provider with “Lawn mowing and gardening services” even if the words are different.
Searching by embeddings is much faster than manually filtering thousands of records.
No matter how many service providers are added, AI-based search remains efficient.
AI-Powered Search API
Now that our service provider data is stored with AI embeddings, we need to build an API that can:
Take a user’s search query (e.g., “I need a licensed electrician in Sydney available this Saturday”)
Convert the query into an embedding (so AI can understand the meaning)
Compare it against stored embeddings in PostgreSQL
Return the most relevant service providers
How Does AI Search Work?
✅ User types a natural language query → The AI converts it into an embedding.
✅ Database searches for the closest matches → It compares the query’s embedding with stored service provider embeddings.
✅ Results are ranked based on similarity → The most relevant providers appear first.
Step 1: Create a Search API in Node.js
Now, let’s write an Express.js API to handle AI-powered search.
const express = require("express"); const { Client } = require("pg"); const { OpenAI } = require("openai"); require("dotenv").config();
const app = express(); const port = 3000; const db = new Client({ connectionString: process.env.DATABASE_URL }); const openai = new OpenAI({ apiKey: process.env.OPENAI_API_KEY });
async function getQueryEmbedding(query) { const response = await openai.embeddings.create({ model: "text-embedding-ada-002", input: [query] }); return response.data[0].embedding; // Convert query into an AI vector }
app.get("/search", async (req, res) => { const { query } = req.query; if (!query) return res.status(400).json({ error: "Query is required" });
await db.connect(); const queryEmbedding = await getQueryEmbedding(query);
const result = await db.query( `SELECT id, name, services, location, work_days, has_insurance, 1 - (embedding <=> $1) AS similarity FROM service_providers ORDER BY similarity DESC LIMIT 5;`, [queryEmbedding] );
await db.end(); res.json({ providers: result.rows }); });
app.listen(port, () => console.log(`Server running on port ${port}`));
So what are we doing here? We are:
Setting up an Express.js API to handle search requests.
Converting user queries into AI embeddings using OpenAI.
Searching PostgreSQL for the most relevant matches using vector comparison (embedding <=> $1).
Sorting results based on similarity so the best matches appear first.
Step 2: Testing the AI Search API
Now, let’s test our AI-powered search by making a request:
curl "http://localhost:3000/search?query=licensed electrician available Saturday in Sydney"
Example Response:
{ "providers": [ { "id": 2, "name": "John Smith", "services": ["Electrician"], "location": "Sydney", "work_days": ["Saturday", "Monday"], "has_insurance": true, "similarity": 0.92 }, { "id": 5, "name": "Sarah Johnson", "services": ["Electrical Repair", "Plumbing"], "location": "Sydney", "work_days": ["Saturday"], "has_insurance": false, "similarity": 0.87 } ] }
Why Is This Powerful?
🚀 A search like “I need someone to fix my wiring this weekend” will find electricians available on Saturday, even if “wiring” isn’t explicitly listed.
🚀 No need for users to manually filter availability, insurance, or location.
🚀 The AI search engine runs efficiently even with thousands of service providers.
Advanced Stuff
Let’s make it even better. A good search system doesn’t just return any results, it should return the best results quickly and accurately.
Improve Relevance with Weighted Scoring
Right now, our search only ranks results by similarity. But what if two providers have the same similarity score? We can boost ranking based on factors like Years of experience, Customer ratings, Response time etc
SELECT id, name, services, location, work_days, has_insurance, (1 - (embedding <=> $1)) * 0.7 + experience * 0.2 + rating * 0.1 AS final_score FROM service_providers ORDER BY final_score DESC LIMIT 5;
Here,
Similarity score (70%): AI-powered match based on query understanding.
Experience (20%): Gives a slight boost to more experienced providers.
Customer rating (10%): Ensures high-quality service providers rank higher.
This makes the search more accurate and ensures better quality providers appear first.
Speed Up Search with Pre-Computed Embeddings
Generating embeddings on the fly can slow down search performance. Instead of computing them every time, we can:
Cache embeddings in Redis or store precomputed embeddings to make search instant.
Batch process new providers by running an embedding update script once per day instead of on every search.
Handle More Complex Queries with Query Refinement.
Sometimes, users type vague or unclear queries (e.g., “I need help with my house.”). AI can rephrase these queries to make them more specific. We can use GPT-4 to refine queries before performing the search.
async function refineQuery(userQuery) { const response = await openai.chat.completions.create({ model: "gpt-4", messages: [ { role: "system", content: "Rephrase this query to make it more specific for a home services search." }, { role: "user", content: userQuery } ] }); return response.choices[0].message.content; }
For example, if a user queries "I need help with my house.", the refined query would say “Looking for a home repair service in Sydney for plumbing and electrical work.”
This makes search smarter and more effective, especially when users aren’t sure how to phrase their requests.
Implement Real-Time Autocomplete for a Better UX
A good search should suggest results as users type. Instead of waiting for a full query, we can provide instant AI-powered suggestions. Using Redis & AI models, we can predict user intent and suggest relevant searches dynamically. This predicts common searches based on past user queries, suggests options before users finish typing, and improves speed and usability of search experience.
const redis = require("redis"); const client = redis.createClient();
async function getSearchSuggestions(input) { const suggestions = await client.lrange(`search_suggestions:${input}`, 0, 5); return suggestions; }
AI-Powered Search is the Future
We just looked at a powerful example, but that's not all. AI-powered search can also be used at:
Healthcare Platforms - “Find me a pediatrician in Melbourne who accepts Medicare and is available on Friday.”
Job Portals - “Looking for a remote frontend developer role with React and at least 3 years of experience.”
E-Commerce Search - “I need a waterproof hiking backpack under $100 with good reviews.”
Real Estate Listings - “Find me a 3-bedroom house in Sydney with a backyard and pet-friendly policy.”
Legal Services - “I need a divorce lawyer who handles child custody cases in New York.”
B2B Supplier Search - “Looking for a bulk organic coffee supplier in Colombia with fair-trade certification.”
If you want to stay ahead in your industry, an AI-driven search experience is no longer a luxury. It’s a necessity. The future of search is here. Are you ready to build it?